I mentioned in my Python Class posts that I really enjoyed the instructor and I planned to eventually move to doing her Web Dev Course. Well, I’ve started working on that one. It’s not a “100 Days of Projects” so I’ve not been quite blitzing through it like I did the Python Course, and it’s a lot of material I am already way more familiar with. Hell, I skipped over the first third of the course because it was the same HTML/CSS content included int he Python Course.
My main goal here, is to get much more familiar with JavaScript. I have dabbled, lightly, in JavaScript (ok, let’s go with JS from here on), and well, it’s a coding language, so I already have a lot of the “logic” side down. At some point, learning new programming languages just becomes about learning the syntax and maybe some of the special uses for a particular language. I’d only say I am Intermediate or above in Python and HTML/PHP, but I’m familiar with several other languages including BASIC, C, C++, LSL (Linden Scripting Language), Arduino (mostly just C), Bash Shell scripting, SQL, Cold Fusion, and probably some other I am forgetting.
It all mostly works the same on the base level, a loop is a loop, conditionals are conditional, and it’s just a matter of remembering if the language uses braces or parenthesis or semi colons and if tabs matter. And that’s where Google becomes your friend.
Anyway, the first real stand along project of this course is a simple Dice rolling game. And I have to say, “game” is the loosest sense of the word. It’s a webpage you refresh to see if Player 1 or Player 2 has the higher dice face. I might actually try to make a more complex version of this later where you select from different Dice and it rolls them, for you know, gaming. Sure there are plenty of D&D Dice roller apps, but it would be a fun exercise.
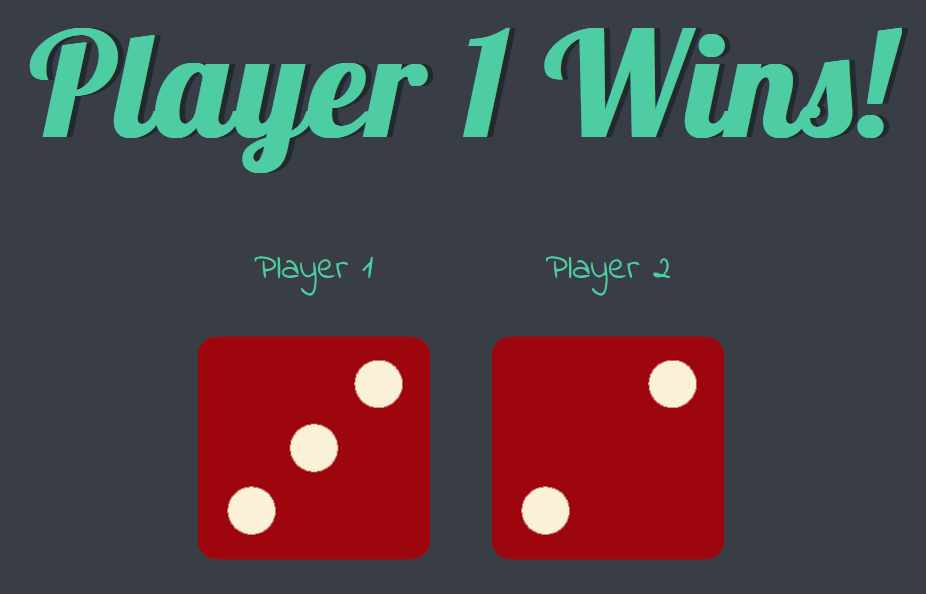
The class provided the basic HTML Structure and graphics, since the focus was on the JavaScript side of things. It’s not an overly complex project, but here is the JS Code.
function setImage(diceImage, roll) {
console.log(roll);
if(roll === 1) { rolled = "images/dice1.png"; }
else if(roll === 2) { rolled = "images/dice2.png"; }
else if(roll === 3) { rolled = "images/dice3.png"; }
else if(roll === 4) { rolled = "images/dice4.png"; }
else if(roll === 5) { rolled = "images/dice5.png"; }
else { rolled = "images/dice6.png"; }
diceImage.src = rolled;
}
let player1Roll = Math.floor(Math.random()*6)+1;
let player2Roll = Math.floor(Math.random()*6)+1;
let dice1Image = document.querySelector("img.img1");
let dice2Image = document.querySelector("img.img2");
if( player1Roll > player2Roll) {
document.getElementById("headtext").textContent = "Player 1 Wins!";
}
else if (player2Roll > player1Roll) {
document.getElementById("headtext").textContent = "Player 2 Wins!";
}
else {
document.getElementById("headtext").textContent = "Its a Tie!";
}
setImage(dice1Image,player1Roll);
setImage(dice2Image,player2Roll);
So what’s the process here. Let’s skip the function at the top for now. Step one is to roll two dice. This is done with Math.random, which selects a random value between 0 and 1. Dice have 6 sides, so we multiply this by 6, to get a number between 0 and 6. But technically it’s a number between 0 and 5.999999~ into infinity, not 6. And Math.floor rounds things down, so it’s actually between 0 and 5. So we need to add one.
let player1Roll = Math.floor(Math.random()*6)+1;
let player2Roll = Math.floor(Math.random()*6)+1;
After this is some selectors to pick the the two dice images off the page, so they can be updated to reflect the rolls later.
let dice1Image = document.querySelector("img.img1");
let dice2Image = document.querySelector("img.img2");
Next we get to use a conditional chain to determine the winner and update the h1 tag on the webpage to display the winner, or if it was a tie. I’m not going to repeat that code here again.
Lastly we make two calls to that function I mentioned before, the one at the top of the script, that updates the image for each dice. The function takes the document selector, and the value of each roll as an input. It runs the roll value (1-6) through a selector and then sets the dice image on the HTML document to the appropriate image.
Could this code all be cleaner, probably, but it gets the job done and is easy to follow. There is probably a simpler way to set the images. The image selector could also just look for 0-5 mapped to 1-6 instead of adding 1. But what if I needed this function to do more based on these rolls. Maybe it becomes part of a larger game and deals damage or something. Personally, this is why I prefer code like this, that isn’t perfectly compact, because it becomes functionally more useful down the road.
Also, the game is available to play at this link.
Josh Miller aka “Ramen Junkie”. I write about my various hobbies here. Mostly coding, photography, and music. Sometimes I just write about life in general. I also post sometimes about toy collecting and video games at Lameazoid.com.